bool stringComplete = false;
char index = 0;
void setup() {
Serial.begin(115200);
delay(500);
Serial.println("IsDelta");
}
void loop() {
if(stringComplete){
sendGcode(index);
stringComplete = false;
index++;
if (index > 4) {
index = 0;
}
}
}
void serialEvent() {
while (Serial.available()) {
char inChar = (char)Serial.read();
if (inChar == '\n') {
stringComplete = true;
}
}
}
void sendGcode(char i){
switch (i) {
case 0: {
Serial.println("G28");
break;
}
case 1: {
Serial.println("G01 Z-280");
break;
}
case 2: {
Serial.println("G01 X160");
break;
}
case 3: {
Serial.println("G02 I-160 J0 X160 Y0");
break;
}
case 4: {
Serial.println("G01 X0");
break;
}
default: break;
}
}
Delta X 2 has 2 Serial ports.

These 2 ports are used to connect with Conveyor or Slider Rail. These are two ports that Delta X 1 does not have.
To enable these two ports to control Conveyor, you use the following command:M330 [R<index>] hoặc M331 [R<index>].
index =
0 : None.
1 : Conveyor.
2 : Slidingrail.
Example (select port 2 for conveyor): M331 R1

You can use these 2 ports to use Arduino to control Delta X 2.
I use Arduino Mega 2560 and use Serial1 to connect to Serial Port 1 of Robot (If you use Arduino Uno then use default Serial).
The connection is as follows: Vcc <--> Vcc, Gnd <--> Gnd, TX <--> RX, RX <--> TX

To enable Serial port on robot by Arduino. Please send the command "IsDelta\n" from Arduino to Robot.
Here is a simple code to control Robot using Serial 1 port of Arduino. (Note that the '\n' after every command sent to the robot is required. If you use the println("IsDelta") function, '\n' is not needed because println("IsDelta") = print("IsDelta\n")
Serial1.begin(115200);
Serial1.println("IsDelta");
delay(100);
Serial1.println("G28");
Serial1.println("G01 Z-350");

If you send too much G-code to the queue of robot, the robot will freeze so you should wait until Robot responds "Ok", send next command.
Here is a basic library that I wrote.
https://github.com/deltaxrobot/Delta-X-Example/tree/master/Delta_X_With_External_Arduino?fbclid=IwAR0Sa9wRxxs7tf_tkwB9J39NPRoSvZyZLjKkssO6oRsH8hpKR6bT2oBgPcU
#include "DeltaXRobot.h"
DeltaXRobot DeltaX;
void setup()
{
Serial.begin(115200);
DeltaX.SetSerial(&Serial1);
DeltaX.Gcode("G28");
DeltaX.Gcode("G01 Z-350");
DeltaX.Gcode("G01 X-100");
DeltaX.Gcode("G02 I100 J0 X-100 Y0");
DeltaX.Gcode("G03 I100 J0 X-100 Y0");
DeltaX.BeginGcode("subprogram1");
DeltaX.Home();
DeltaX.MoveZ(-300);
DeltaX.MoveZ(-270);
DeltaX.MoveZ(-300);
DeltaX.MoveZ(-270);
DeltaX.MoveZ(-300);
DeltaX.MoveZ(-270);
DeltaX.EndGcode();
DeltaX.BeginGcode("subprogram2");
DeltaX.Home();
DeltaX.MoveZ(-300);
DeltaX.MoveXY(100,100);
DeltaX.MoveXY(-100, 100);
DeltaX.MoveXY(-100, -100);
DeltaX.MoveXY(100, -100);
DeltaX.Gcode("G01 X0 Y0");
DeltaX.Gcode("G01 Z-330");
DeltaX.EndGcode();
DeltaX.Run("subprogram1");
}
void loop()
{
DeltaX.Execute();
if (DeltaX.IsAllStop() == true && DeltaX.IsSelectingProgram("subprogram1") == true)
{
DeltaX.Run("subprogram2");
}
}
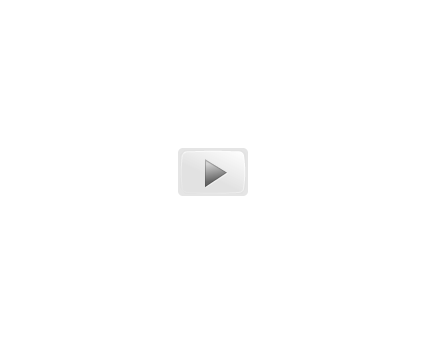